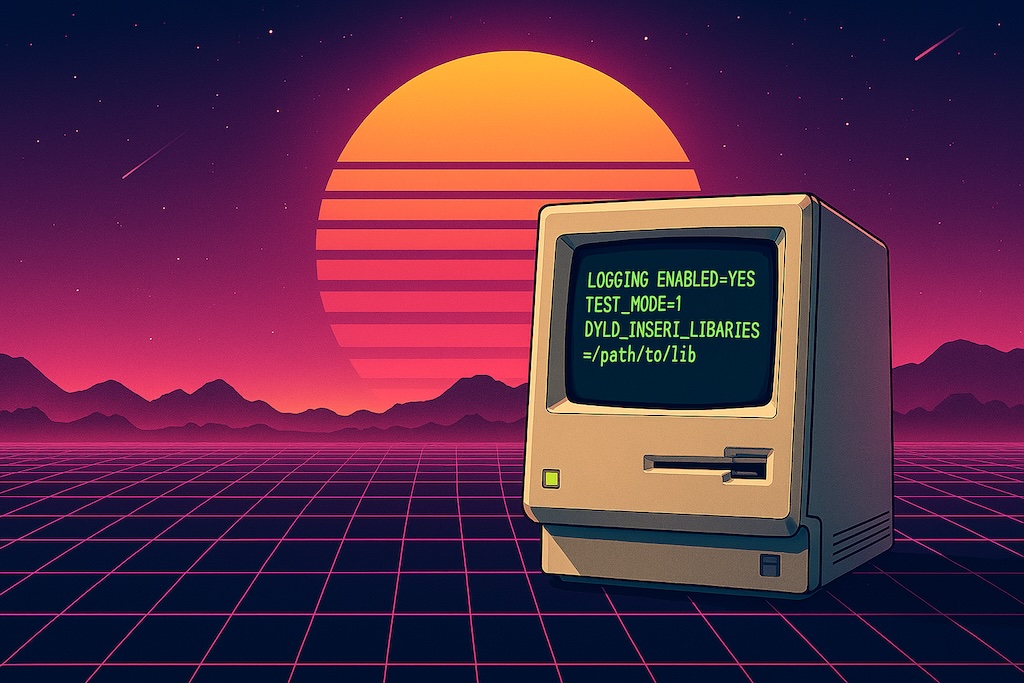
The Ultimate Guide to Xcode Environment Variables
Environment variables are powerful, often overlooked tools that can shape the way your iOS app behaves at runtime. With proper usage, you can control logging, test modes, and even the dynamic linking process—all without having to rebuild your application each time. This post compiles all the important Xcode environment variables you should know about, including both system-level and DYLD (Dynamic Linker) variables.
Table of Contents
- What Are Environment Variables?
- Configuring Environment Variables in Xcode
- Common System Environment Variables
- DYLD Environment Variables
- Best Practices
- Conclusion
What Are Environment Variables?
In simple terms, environment variables are key-value pairs that influence the runtime behavior of your application. They come in handy for:
- Debugging: Toggling verbose logs or specialized checks.
- Testing: Simulating different configurations (e.g., test endpoints or feature flags).
- Performance Analysis: Monitoring how your app starts up and loads frameworks.
Because these variables don’t require code changes, they’re incredibly convenient for iterative development and QA testing.
Configuring Environment Variables in Xcode
- Open Your Scheme
- Select your project in the Navigator, then choose Edit Scheme….
- Select the Run Section
- In the left sidebar, choose the Run option under your scheme.
- Go to Arguments
- Switch to the Arguments tab. You’ll see two areas:
- Arguments Passed On Launch: Flags appended to the launch command.
- Environment Variables: Key-value pairs available to your app at runtime.
- Switch to the Arguments tab. You’ll see two areas:
- Add a New Environment Variable
- Click the + button under Environment Variables.
- Enter the name of your variable (e.g.,
OS_ACTIVITY_MODE
) and its value (e.g.,disable
). - Ensure the checkbox is checked so it’s active during launch.
Common System Environment Variables
Below are some frequently used environment variables in iOS projects.
OS_ACTIVITY_MODE
- Purpose: Controls how much log output is shown from Apple’s unified logging system (
os_log
). - Values:
default
– Normal logging.disable
– Turns off all logging output.
- Why Use It?
- Helps declutter your Xcode console by eliminating system logs you don’t need during debugging.
CFNETWORK_DIAGNOSTICS
- Purpose: Enables detailed logging of network activities at a low level.
- Value: Set to
1
to activate. - Why Use It?
- Troubleshoot complex networking issues, especially if you suspect certain requests are failing silently.
NSDebugEnabled
- Purpose: Activates additional internal debug checks within Foundation.
- Value:
YES
to enable. - Why Use It?
- Helpful for deep debugging in complex Foundation-based logic or custom classes.
UI_TEST_MODE (Custom)
- Purpose: A custom variable commonly used to distinguish between normal runs and UI test runs.
- Value:
true
orfalse
. - Why Use It?
- Allows you to bypass login flows, disable animations, or otherwise customize the UI for automated testing.
- Access it via
ProcessInfo.processInfo.environment["UI_TEST_MODE"]
in Swift.
DYLD Environment Variables
DYLD environment variables instruct the dynamic linker on how to locate and load libraries or frameworks. While many are restricted on physical iOS devices for security reasons, they can still be powerful when debugging in the iOS Simulator or on macOS.
DYLD_LIBRARY_PATH
- Purpose: Tells the dynamic linker where to look for libraries before the default system library paths.
- Value: A colon-separated list of directories.
- Example:
/Users/username/libs:/usr/local/lib
.
- Example:
- Why Use It?
- Load a custom or modified version of a library to test how it behaves in your app.
DYLD_FRAMEWORK_PATH
- Purpose: Similar to
DYLD_LIBRARY_PATH
, but specifically for frameworks (.framework
bundles). - Value: A colon-separated list of directories.
- Example:
/Users/username/Frameworks
.
- Example:
- Why Use It?
- Override the path to frameworks, letting you experiment with different versions during development.
DYLD_INSERT_LIBRARIES
- Purpose: Inject one or more dynamic libraries into your app’s process at launch.
- Value: A colon-separated list of library paths.
- Example:
/path/to/customLibrary.dylib:/another/lib.dylib
.
- Example:
- Why Use It?
- Useful for advanced debugging or hooking in libraries.
- Note: Generally restricted on physical iOS devices, so it’s most effective on the simulator.
DYLD_IMAGE_SUFFIX
- Purpose: Appends a suffix to library or framework names when they are loaded.
- Value: A string suffix.
- Example:
_debug
so thatUIKit
becomesUIKit_debug
.
- Example:
- Why Use It?
- If you maintain separate debug builds of frameworks, you can automatically pick the debug versions for testing.
DYLD_PRINT_STATISTICS
- Purpose: Prints detailed statistics about the app’s launch process, including load times and memory usage of linked libraries.
- Value:
1
(or any non-empty string). - Why Use It?
- Gain insight into how fast your app is loading frameworks and identify possible bottlenecks.
- Example output might show total pre-main time, rebase/bind times, and the number of images loaded.
Best Practices
- Avoid Storing Sensitive Data
- Don’t include API keys or tokens in environment variables within your Xcode scheme. Use a secure storage or dedicated configuration management solution.
- Use Descriptive Names
- Variables like
DEBUG_VERBOSE_LOGGING
orAPI_ENDPOINT_URL
make it clear what they control.
- Variables like
- Leverage
ProcessInfo
- Read environment variables from Swift via
ProcessInfo.processInfo.environment["VARIABLE_NAME"]
. This way, you can programmatically enable or disable features at runtime.
- Read environment variables from Swift via
- Mind the Checkboxes
- Always verify the environment variables are checked in the scheme; otherwise, they won’t take effect.
- Share Schemes Wisely
- If your Xcode schemes are version-controlled, ensure you don’t accidentally commit sensitive or local paths.
- Remember iOS Device Restrictions
- Most DYLD variables won’t work on a physical iPhone or iPad due to security constraints. Use them primarily on the simulator or macOS.
Conclusion
Environment variables—both standard and DYLD—are integral to tailoring your app’s behavior for debugging, testing, and performance analysis. Whether you’re reducing console clutter with OS_ACTIVITY_MODE
, diagnosing networking with CFNETWORK_DIAGNOSTICS
, or investigating load times with DYLD_PRINT_STATISTICS
, these tools can save you time and headaches in the long run.
Have a favorite environment variable trick or a unique setup? Feel free to share your experiences—I’m always eager to learn new ways to make development faster, safer, and more efficient!